반응형
이미지 파일 처리
함수 설명
cv2.imread(filename[, flags]) >> retval
|
||||
인수 설명 |
|
cv2.imwrite(filename, img[, params]) >> retval
|
|||||
인수 설명 |
|
영상파일 읽기
소스 코드
import cv2
def print_matInfo(name, image):
if image.dtype == 'uint8': mat_type = 'CV_8U'
elif image.dtype == 'int8': mat_type = 'cv_8S'
elif image.dtype == 'uint16': mat_type = 'cv_16U'
elif image.dtype == 'int16': mat_type = 'cv_16S'
elif image.dtype == 'float32': mat_type = 'cv_32F'
elif image.dtype == 'float64': mat_type = 'cv_64F'
nchannel = 3 if image.ndim == 3 else 1
print("%12s: depth(%s), channels(%s) -> mat_type(%sC%d)"
% (name, image.dtype, nchannel, mat_type, nchannel))
title1, title2 = "gray2gray", "gray2color"
gray2gray = cv2.imread("cat.jpg", cv2.IMREAD_GRAYSCALE)
gray2color = cv2.imread("cat.jpg", cv2.IMREAD_COLOR)
if gray2gray is None or gray2color is None :
raise Exception("영상파일 읽기 에러")
print("행렬 좌표 (100, 100) 화소값")
print("%s %s"% (title1, gray2gray[100, 100]))
print("%s %s\n" % (title2, gray2color[100, 100]))
print_matInfo(title1, gray2gray)
print_matInfo(title2, gray2color)
cv2.imshow(title1, gray2gray)
cv2.imshow(title2, gray2color)
cv2.waitKey(0)
cv2.destroyAllWindows()
실행 결과
행렬 좌표 (100, 100) 화소값
gray2gray 110
gray2color [ 53 118 116]
gray2gray: depth(uint8), channels(1) -> mat_type(CV_8UC1)
gray2color: depth(uint8), channels(3) -> mat_type(CV_8UC3)
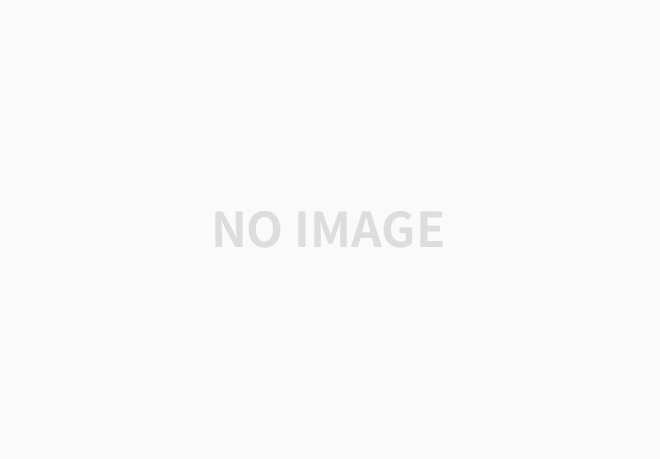
영상파일 저장
소스 코드
import numpy as np
import cv2
image = cv2.imread("cat.jpg", cv2.IMREAD_COLOR)
if image is None: raise Exception("영상처리 읽기 에러")
params_jpg = (cv2.IMWRITE_JPEG_QUALITY,10)
params_png = [cv2.IMWRITE_PNG_COMPRESSION,9]
cv2.imwrite("write_test1.jpg", image)
cv2.imwrite("write_test2.jpg", image,params_jpg)
cv2.imwrite("write_test3.png", image,params_png)
cv2.imwrite("write_test4.bmp", image)
print("저장 완료")
실행 결과
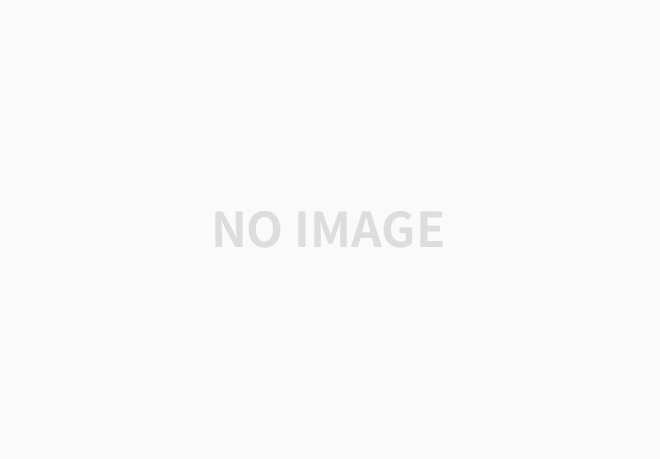
비디오파일 처리
VideoCapture 클래스
cv2.VideoCapture() >> <VideoCapture object> cv2.VideoCapture(filename) >> <VideoCapture object> cv2.VideoCapture(device) >> <VideoCapture object>
|
|||||||||
인수 설명 |
|
cv2.VideoCapture.open(filename) >> retval cv2.VideoCapture.open(device) >> retval
|
cv2.VideoCapture.isOpened() >> retval
|
cv2.VideoCapture.release() >> None
|
cv2.VideoCapture.get(propld) >> retval
|
|||||||||
인수 설명 |
|
cv2.VideoCapture.set(propld, value) >> retval
|
|||||||||
인수 설명 |
|
cv2.VideoCapture.release() >> None
|
retrueve([, image[, flag]]) >> retval, image
|
|||||||||
인수 설명 |
|
cv2.VideoCapture.read([image]) >> retval, image
|
VideoWriter 클래스
cv2.VideoWriter([filename, fourcc, fps, frameSize[, isColor]]) >> <VideoWriter object> cv2.VideoWriter.open(filename, fourcc, fps, frameSize[, isColor]) >> retval |
|||||||||
인수 설명 |
|
cv2.VideoWriter.isOpened() >>retval
|
cv2.VideoWriter.write(image) >> None
|
cv2.VideoWriter.isOpen()
|
cv2.VideoWriter.isOpened()
|
소스 코드
import cv2
capture = cv2.VideoCapture("catvideo.mp4")
if capture.isOpened() == False:
raise Exception("카메라 연결 안됨")
while True:
ret, frame = capture.read()
if not ret: break
if cv2.waitKey(30) >= 0: break
cv2.imshow('frame', frame)
capture.release()
실행 결과(동영상 캡처본)
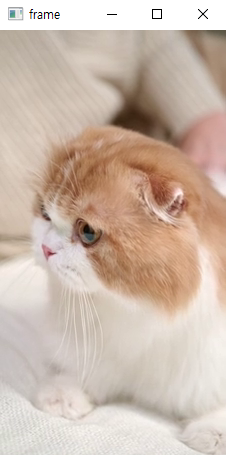
웹캠을 사용하여 코드를 작성할 경우에는
import cv2
capture = cv2.VideoCapture(장치 번호)
if capture.isOpened() == False:
raise Exception("카메라 연결 안됨")
while True:
ret, frame = capture.read()
if not ret: break
if cv2.waitKey(30) >= 0: break
cv2.imshow('frame', frame)
capture.release()
장치 번호를 VideoCapture()에 입력해야 한다.
반응형
'영상처리 프로그래밍 > OpenCV-Python' 카테고리의 다른 글
관심 영역(ROI) (0) | 2022.11.16 |
---|---|
논리(비트) 연산 (0) | 2022.11.16 |
OpenCV 채널 처리 함수 (0) | 2022.11.03 |